Integrating APIs with Flutter: A Comprehensive Guide
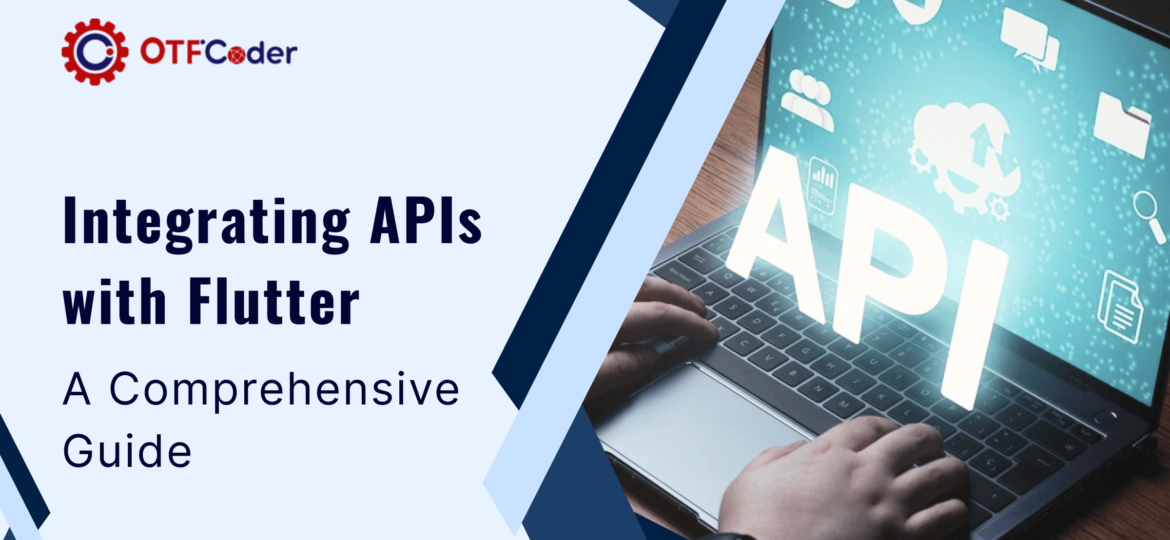
In the fast-evolving world of mobile app development, creating applications that seamlessly interact with external services is crucial. APIs (Application Programming Interfaces) enable this interaction, allowing apps to access external data and functionalities.
Introduction to API Integration in Flutter
Flutter’s growing popularity among developers is due to its expressive and flexible UI, high performance, and single codebase for multiple platforms. However, the true power of any app lies in its ability to connect with various services, which is where APIs come into play. Whether you are looking to hire Flutter developers or want to enhance your own skills, understanding API integration is essential.
Why API Integration is Important
API integration allows your Flutter app to:
– Fetch real-time data from servers.
– Connect with third-party services (e.g., social media, payment gateways).
– Enhance functionality without extensive code.
– Provide a richer user experience by leveraging external resources.
Step-by-Step Guide to API Integration in Flutter
1. Setting Up Your Flutter Project
Create a new Flutter project or open an existing one where you plan to integrate the API.
“`bash
flutter create api_integration_example
cd api_integration_example
“`
2. Adding Dependencies
Add HTTP requests by adding the `http` package to your `pubspec.yaml` file.
“`yaml
dependencies:
flutter:
sdk: flutter
http: ^0.13.3
“`
3. Making HTTP Requests
Create a service class to manage your API calls. For example, to fetch data from a sample API, you can create a `api_service.dart` file.
“`dart
import ‘package:http/http.dart’ as http;
import ‘dart:convert’;
class ApiService {
final String apiUrl = “https://jsonplaceholder.typicode.com/posts“;
Future<List<dynamic>> fetchPosts() async {
final response = await http.get(Uri.parse(apiUrl));
if (response.statusCode == 200) {
return json.decode(response.body);
} else {
throw Exception(‘Failed to load posts’);
}
}
}
“`
4. Displaying Data in the UI
Use the `ApiService` class to fetch data and display it in your Flutter app. Modify your `main.dart` to include the API call and display the data.
“`dart
import ‘package:flutter/material.dart’;
import ‘api_service.dart’;
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: PostListScreen(),
);
}
}
class PostListScreen extends StatefulWidget {
@override
_PostListScreenState createState() => _PostListScreenState();
}
class _PostListScreenState extends State<PostListScreen> {
late Future<List<dynamic>> posts;
@override
void initState() {
super.initState();
posts = ApiService().fetchPosts();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(‘Posts’),
),
body: FutureBuilder<List<dynamic>>(
future: posts,
builder: (context, snapshot) {
if (snapshot.connectionState == ConnectionState.waiting) {
return Center(child: CircularProgressIndicator());
} else if (snapshot.hasError) {
return Center(child: Text(‘Error: ${snapshot.error}’));
} else {
return ListView.builder(
itemCount: snapshot.data?.length,
itemBuilder: (context, index) {
return ListTile(
title: Text(snapshot.data?[index][‘title’]),
subtitle: Text(snapshot.data?[index][‘body’]),
);
},
);
}
},
),
);
}
}
“`
5. Handling Errors and Edge Cases
Effective API integration involves robust error handling. Ensure your app gracefully handles scenarios such as network failures, invalid responses, and timeouts.
“`dart
Future<List<dynamic>> fetchPosts() async {
try {
final response = await http.get(Uri.parse(apiUrl));
if (response.statusCode == 200) {
return json.decode(response.body);
} else {
throw Exception(‘Failed to load posts’);
}
} catch (e) {
throw Exception(‘Failed to load posts: $e’);
}
}
“`
6. Optimizing Performance
For a professional Flutter development approach, consider optimizing API calls by:
– Caching responses to reduce redundant network requests.
– Using efficient JSON parsing methods.
– Implementing pagination for large data sets.
Conclusion
Integrating APIs with Flutter is a powerful way to enhance your app’s functionality and provide a richer user experience. Whether you are a developer looking to expand your skills or a business aiming to hire Flutter developers, mastering API integration is crucial. By following this comprehensive guide, you can ensure your Flutter applications are well-equipped to connect with external services seamlessly. Hire professional Flutter developers to help you integrate APIs efficiently and elevate your app’s performance. Contact us today to learn more about our Flutter development services and how we can assist you in building robust, high-performing applications.