Laravel Validation – A Detailed Guide For Secure And Efficient Data Handling
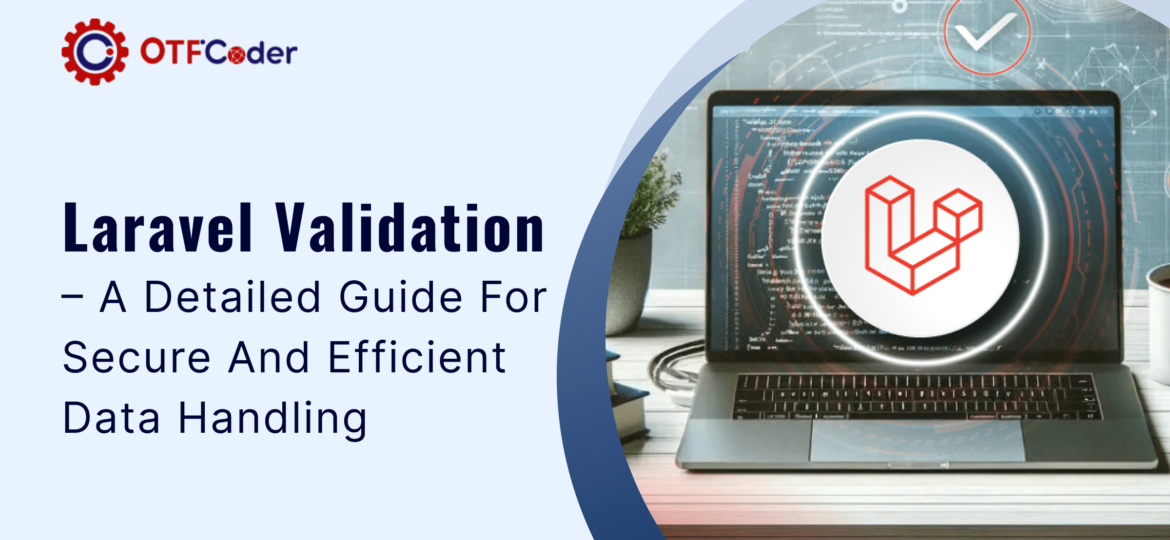
In the world of web development, data validation is crucial for maintaining the integrity and security of your applications. Laravel, one of the most popular PHP frameworks, offers robust and flexible validation features that are essential for any developer aiming to build secure and efficient applications. This blog explores the intricacies of Laravel validation, providing a detailed guide on how to utilize these features effectively. Laravel validation is designed to protect your applications from inconsistencies and security vulnerabilities by ensuring that the data your users input meets your specified criteria before it’s processed or stored. By leveraging Laravel’s validation rules, developers can easily enforce various constraints on data, such as data type, size, format, and uniqueness, which helps prevent common security risks like SQL injections and data corruption.
Why Use Laravel for Validation?
Choosing Laravel for data validation comes with numerous benefits:
Comprehensive Rule Set
The comprehensive set of validation rules in Laravel not only addresses basic data integrity but also extends to more nuanced aspects of data handling. This robust collection includes validations for confirming array types, managing multiple dimensions with arrays.*, and ensuring the presence of values with exist or are unique. Additionally, Laravel supports the direct extension of functionality through custom rule objects, allowing developers to tailor the validation logic to specific application needs. This makes Laravel’s validation suite exceptionally adaptable, capable of handling virtually any user input validation scenario that modern web applications might encounter. For developers looking to go beyond the conventional, Laravel’s ability to integrate with custom rules seamlessly is a game-changer, providing both flexibility and power in maintaining data integrity and user experience.
Ease of Use
Implementing validation in Laravel is straightforward, thanks to its fluent syntax and variety of helper functions. Validators can be triggered easily, and custom error messages can be set up to inform users about validation failures. The framework’s built-in validation functionality is integrated directly with the Laravel service container, allowing for dependency injection and making the validation process highly flexible. Additionally, Laravel provides several different ways to trigger validation, including form requests, manual creation of validator instances, or even inline within controller actions. This versatility ensures that regardless of your application’s structure, you can implement validation seamlessly. Laravel enhances the user experience by employing robust error handling techniques that automatically navigate users back to their original input form, highlighting errors clearly. This immediate, transparent feedback helps users easily identify and correct their errors, streamlining the data submission process.
Extensibility
Laravel’s extensibility in validation rules allows developers to not only tweak existing validations but also to craft entirely bespoke validators that align perfectly with unique project demands. This flexibility is particularly beneficial in complex application ecosystems where standard validation parameters may not suffice. Developers can harness Laravel’s powerful service container to inject custom validation services or utilize the framework’s extensive events and listeners to perform validation under specific application states. Furthermore, Laravel’s ability to integrate with various third-party packages enables developers to incorporate sophisticated validation techniques that can handle internationalization, multi-form processes, and asynchronous validations, enhancing the application’s scalability and responsiveness to diverse user requirements.
Automatic Redirection
In Laravel, the automatic redirection feature for web forms is not only about improving user experience by providing quick feedback on errors but also enhances the overall flow of data entry processes. This functionality simplifies the development of user-friendly forms by eliminating the need for additional code to manage redirection and error handling manually. Moreover, it ensures that user inputs are retained, so they don’t have to re-enter all data upon encountering validation errors, reducing frustration and increasing the likelihood of successful form submission. This feature is especially beneficial in complex forms where multiple fields require validation, as it helps maintain the context of user inputs and errors, guiding users more clearly on how to correct their submissions effectively.
Implementing Validation in Laravel
In Laravel, validation is typically facilitated through the Validator facade or by utilizing the validate method within controllers. Here’s a basic example of how to validate user input in a form:
php
Copy code
$request->validate([
‘name’ => ‘required|max:255’,
’email’ => ‘required|email|unique:users’,
‘age’ => ‘required|numeric|between:18,65’
]);
This code snippet ensures that the name is required and does not exceed 255 characters, the email is also required, must be unique across the users’ table, and must be a valid email format, and the age must be a number between 18 and 65.
Advanced Validation Scenarios
Laravel’s validation system can handle more complex scenarios using conditional rules, custom validation messages, and even creating custom validation rules. For example, conditional rules can be used to require certain fields only if another field has a specific value, which is particularly useful in complex forms.
Best Practices for Laravel Validation
– Centralize Validation Rules: Keeping validation rules consistent across your application helps maintain code cleanliness and understandability.
– Use Form Request Validation: For complex forms, use form request classes to encapsulate validation rules.
– Custom Error Messages: Customize error messages to make them more user-friendly and helpful.
Conclusion
Laravel validation offers a powerful toolset for developers to ensure data integrity and security in their applications. By understanding and implementing the right validation practices as outlined in this guide, developers can greatly enhance the robustness and user experience of their Laravel applications. Whether you’re a seasoned Laravel developer or new to the framework, mastering Laravel validation is a key skill that will aid in the creation of more secure, efficient, and reliable web applications.