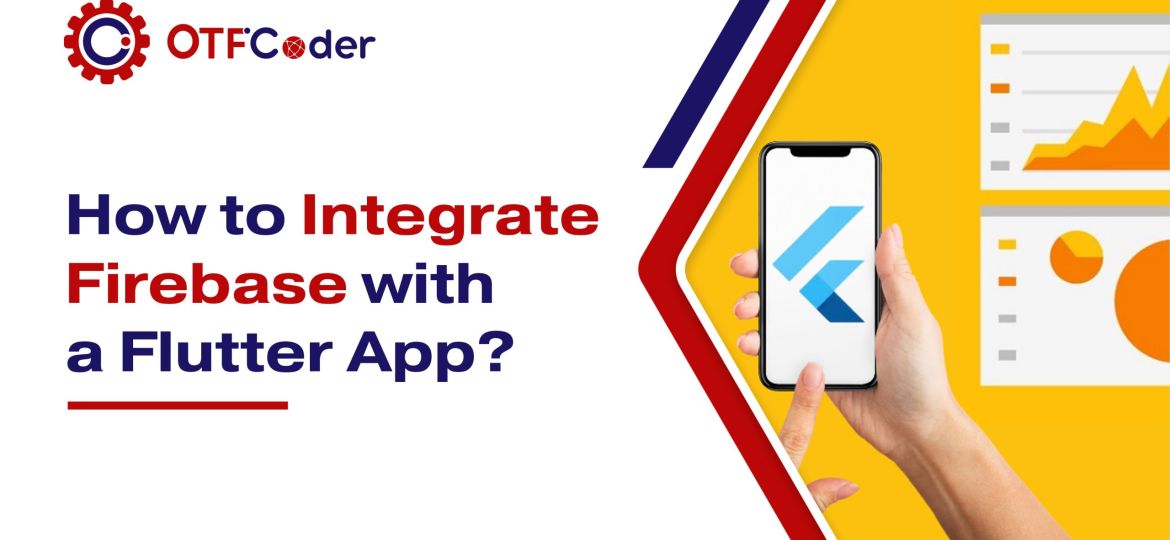
Imagine building a Flutter app that scales effortlessly with real-time database sync, authentication, and cloud functions—all in just a few lines of code! That’s the magic of Firebase!
What is Firebase?
Firebase is a Google backend-as-a-service (BaaS) platform that provides cloud-based services for app development. It enables developers to add authentication, databases, cloud functions, and analytics without managing a separate backend.
Why Use Firebase with Flutter?
- Seamless Integration
Firebase offers official Flutter plugins (FlutterFire), making connecting and using its services easy. This means less setup and more time focusing on your app. There is no need for complex backend coding—Firebase handles it for you.
- Cross-Platform Support
Firebase works smoothly on Android, iOS, and the web. You can build your app once and run it on multiple devices, saving time and effort compared to managing separate backends for each platform.
- Scalability
Whether you have ten users or ten million, Firebase can handle the load. It scales automatically, so you don’t have to worry about managing servers or performance issues. This is perfect for growing apps.
- Rich Features
Firebase offers a wide range of tools, such as authentication, databases, cloud functions, and analytics. These features help you build a complete app without third-party services. Everything is available in one place.
- Google’s Backing
As a Google product, Firebase is reliable, secure, and constantly improving. It offers access to the latest technology, best practices, and strong community support, making It a trusted choice for developers.
Key Features Firebase Offers
- Authentication
Adding user sign-in choices like Google, Facebook, and email/password is simple with Firebase. It manages user authentication securely, so you don’t have to build login systems from scratch. It also includes features like password reset and user verification.
- Firestore & Real-time Database
These are cloud-hosted NoSQL databases that store and sync data in real-time. Firestore is excellent for complex queries, while Real-time Database is ideal for instant updates. Both allow offline access, so users can still interact with the app without the internet.
- Cloud Functions
With Cloud Functions, you can run backend code without setting up servers. Events like user sign-ups or database updates can trigger these functions, helping automate processes and keep your app lightweight.
- Cloud Messaging (FCM)
Firebase Cloud Messaging lets you send push notifications to users. You can notify them about updates, new messages, or promotions. It works across different platforms and improves user engagement.
- Analytics
Firebase provides free analytics to track user behaviour, app performance, and engagement. You can see which features users like the most and where they drop off. This helps you improve your app and grow your audience.
Comparison: Firebase vs. AWS Amplify vs. Supabase
Feature | Firebase | AWS Amplify | Supabase |
Auth Providers | |||
Real-time Sync | |||
Cloud Functions | |||
Hosting | |||
Free Tier |
Firebase is often the easiest and most effective choice for fast, scalable mobile apps!
Step 1: Setting Up the Firebase Project in the Firebase Console
First things first—Let’s set up Firebase like a pro!
- Go to the Firebase Console.
- Click on “Add Project” (This is where Firebase stores app data and configurations).
- Provide a meaningful Project Name.
- Disable “Enable Google Analytics” (unless needed for tracking app performance).
- Click “Create Project” and wait for Firebase to configure.
- Click “Continue” and select the “Flutter” option.
Pro Tip: Always use a structured naming convention, especially if handling multiple apps.
Step 2: Installing Required Dependencies
Before you fly, check if you’ve got the right tools!
Tools You Need:
- Flutter SDK (Ensure it’s updated).
- Firebase CLI (Command-line tool for Firebase).
- FlutterFire CLI (For seamless Firebase integration).
Install FlutterFire CLI:
dart pub global activate flutterfire_cli
Verify Installation:
flutterfire –version
Why use FlutterFire CLI?
- Automates Firebase setup.
- Avoids manual platform-specific configuration.
Step 3: Connecting Firebase to Flutter
Let’s make the magic happen by linking your app with Firebase!
- Login to Firebase in Terminal
- Enter your Firebase email and password.
- Run FlutterFire Configure to Select Firebase Project:
flutterfire configure
Lists all Firebase projects.
Select the one you created.
Choose platforms (Android, iOS, Web, macOS) using arrow keys + space bar.
What happens in the backend?
- Creates firebase_options.dart, which stores Firebase credentials.
- Auto-configures Firebase services.
Step 4: Adding Firebase SDK to Flutter
Time to install Firebase packages!
- Install Core Firebase Packages:
flutter pub add firebase_core firebase_auth
- Import Firebase in main. Dart:
import ‘package:firebase_core/firebase_core.dart’;
import ‘package:firebase_auth/firebase_auth.dart’;
void main() async {
WidgetsFlutterBinding.ensureInitialized();
await Firebase.initializeApp();
runApp(MyApp());
}
Why use WidgetsFlutterBinding.ensureInitialized()?
- Ensures Firebase initializes before the app starts.
- Prevents MissingPluginException errors.
Step 5: Verifying Firebase Connection
Is Firebase working? Let’s check!
- Run your app:
flutter run
- Check the console for the Firebase Initialization Success Message.
- No errors? Congratulations! Firebase is now integrated!
Bonus: Quick Firebase Authentication Test
FirebaseAuth.instance.signInAnonymously().then((user) {
print(“Signed in: ${user.user!.uid}”);
});
Common Issues & Troubleshooting
Oops! Something went wrong? Let’s fix it!
- FlutterFire CLI not found?
flutterfire –version
Restart the terminal if needed.
- Firebase SDK version mismatch?
Flutter Pub outdated
Flutter Pub upgrade
- Project not found in Firebase? Ensure the correct Google account is logged in.
Summing Up: What’s Next?
You did it! Now what?
Next Steps:
- Integrate Firebase Authentication (Google Sign-In, Email/Password).
- Use the Firestore Database to store user data.
- Implement Firebase Push Notifications (FCM).
- Deploy your Flutter app with Firebase Hosting (for web).
Want to take your app to the next level? Drop a comment below on what Firebase feature you want to learn next!
What’s your favourite Firebase feature? Drop your thoughts in the comments!