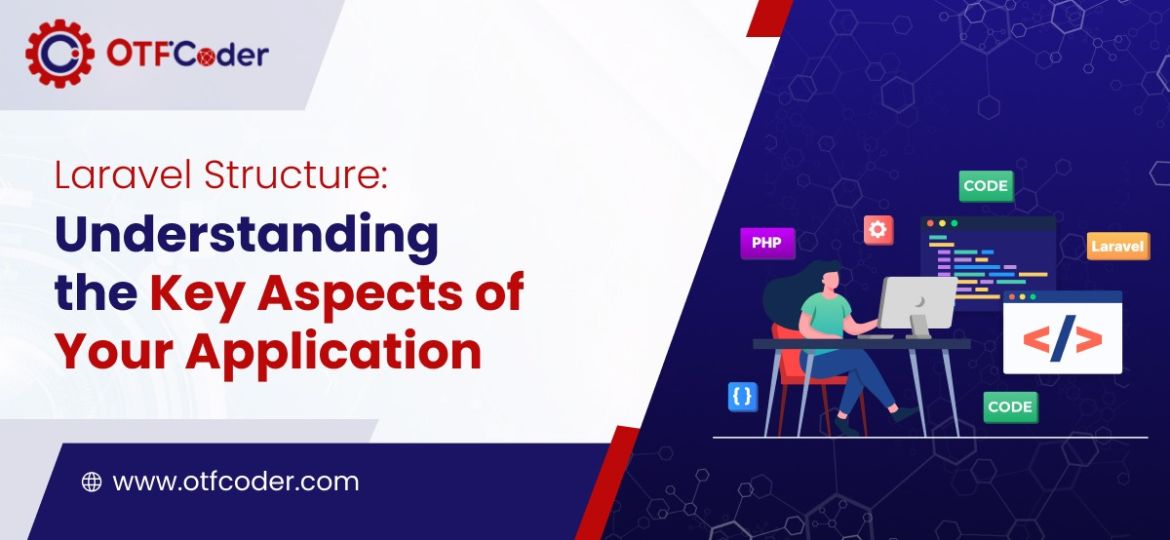
Have you ever attempted putting together furniture without a set of instructions? Missing parts, incorrect screws, and a great deal of wasted time make it a complete pain. It feels precisely the same to code without a transparent organization.
The good news is that everything is kept tidy, easy, and stress-free by the Laravel framework, which functions similarly to a step-by-step manual. Building apps becomes less chore and more enjoyable with Laravel’s unique features, straightforward file setup, and simple MVC design. It keeps you from getting caught up in the commotion and helps you concentrate on making something extraordinary.
Let’s break it down and see why it’s the go-to choice for smooth, efficient web development.
The Foundation: Understanding Laravel’s Core Philosophy
At its heart, Laravel is all about simplicity, modularity, and organization. Built on the MVC (Model-View-Controller) pattern, it gives developers a clear roadmap for structuring code.
Think of the MVC architecture as an online shopping platform. Behind the scenes, the Model manages information such as user accounts, orders, and product details. The stunning shop you see, which uses HTML, CSS, and JavaScript to display products, is called The View. The Controller makes sure that consumer activities, like adding items to a cart, are processed efficiently, acting as the equivalent of the shop’s clerk.
This clear division of roles makes Laravel’s structure easy to understand.
Key Folders and Their Purpose
Getting around the Laravel file organization is similar to entering a neat workplace. Each framework has a distinct function, which improves the development’s effectiveness and appeal.
Think of the structure as a well-organized office:
- App/: This is the main office where work happens. It houses your Models, Controllers, and Middleware and handles all the logic and functionality.
- Routes/ are the pathways leading to different departments. Use web.php for user-facing routes and API.php for backend APIs.
- Resources/ are the presentation materials displayed to clients. It’s what your users interact with.
- Config/: The control room. Manage your app settings here, like database connections and time zones.
- Database/: The archive room for migrations, seeders, and everything database-related.
- Public/: Your front door—users access images, CSS, and the index.php file directly.
- Storage/: The storage room. Logs, backups, and session data are all tucked away here.
Benefits of Laravel’s Structure
Why is Laravel’s structure so important? Let’s take a look.
- Easy Navigation:
A well-organized toolbox is how Laravel’s file structure looks. Because everything is exactly where you expect it to be, you won’t have to waste time searching for a file. Do you require a model to be added? Finalized. Do you wish to alter the view or the route? Everything is in its proper place.
You can focus on designing amazing features instead of trying to find hidden features with this simple, elegant setup. Similar to a map on your app, you’ll always know where to go because of it.
- MVC Architecture:
The MVC architecture separates concerns, which helps you write more manageable and reusable code. Your Controllers handle logic, Views control display, and Models handle data. Because of this apparent division, improvements made to one layer won’t impact the others, keeping your app neat and expandable.
- Modularity:
Laravel’s modular structure allows you to add or remove features without breaking the app. Think of it like building blocks—each piece works independently yet fits perfectly into the whole. Whether you’re integrating a new API or adding a feature, you can do so with minimal fuss and maximum flexibility.
- Built-in Security:
With features like password hashing to protect user data and CSRF protection to stop unwanted operations, Laravel takes security very seriously. As a gatekeeper, middleware gives your application an additional degree of security. With these framework-integrated technologies, you can concentrate on development while Laravel takes care of security.
As we often say, “Laravel’s structure isn’t just a framework—it’s a lifestyle for developers!” It streamlines the entire process, leaving you more time to create and innovate.
Practical Example: How Laravel Structure Works in Action
- Use” Visits a Route: When someone visits /posts, the routes folder directs this request to a controller.
php
Route::get(‘/posts’, [PostController::class, ‘index’]);
- Controller Handles Request: The Controller fetches data from the Model and passes it to the View.
php
public function index() {
$posts = Post::all();
return view(‘posts.index’, compact(‘posts’));
}
- Model Fetches Data: The Model interacts with the database, retrieving blog posts.
php
class Post extends Model {
protected $fillable = [‘title’, ‘content’];
}
- View Displays Content: Finally, the View presents the blog post’ to t’e’ ser in ‘clean, readable format.
blade
@foreach ($posts as $post)
<h2>{{ $post->title }}</h2>
<p>{{ $post->content }}</p>
@endforeach
Advanced Insights: How Laravel’s Structure Boosts Development
For experienced developers, the Laravel framework is a toolbox full with all the necessary tools. It makes difficult jobs fun and manageable. Web development has been raised to a new level through its performance optimization strategies, modular architecture, and integration possibilities.
- Modular Structure
Laravel’s modular packaging system allows you to break your application into distinct, self-contained modules. Each module can handle specific features or domains, making it easier to maintain and scale your application as it grows.
Think about it—every module can have its business logic, models, controllers, and services, keeping your code clean and organized. Collaboration is also made easier by this division of responsibilities since team members can work on distinct modules without stomping on each other’s toes.
- Integration Capabilities
One of the standout Laravel features is its ability to integrate seamlessly with various technologies. Whether you’re adding AJAX for dynamic and responsive applications or building secure RESTful API, Laravel has you covered.
Its routing and controller mechanisms make working with AJAX straightforward. At the same time, its robust API development tools ensure your application remains scalable and secure. This boosts productivity and enhances the user experience, making your applications more interactive and efficient.
- Performance Optimization
Laravel’s structure provides a number of built-in performance techniques to guarantee Laravel’s application functions properly:
- Caching: Laravel supports caching backends like Redis and Memcached. Caching reduces database load and significantly improves response times.
- Database Indexes: Proper indexing of database tables enhances query performance, especially when working with large datasets.
- Query Optimization: Using Eloquent ORM’s sophisticated features and crafting effective queries guarantees quicker data retrieval and more seamless page loads.
Security in Laravel: Protecting Your Application
It is impossible to overestimate the significance of security in web development. Thankfully, the Laravel framework offers robust protections to keep these risks out of your applications.
The following are some ways that Laravel’s built-in capabilities protect your app:
- CSRF Protection
Laravel basically generates a CSRF (Cross-Site Request Forgery) token for every active user session. This token guarantees that the program can only receive requests from authorized users. It guards against unwanted activity by being validated by middleware and inserted in forms.
The csrf_token helper function or the session can be used to retrieve the CSRF token. This adds an additional layer of security to your application by protecting each POST, PUT, PATCH, and DELETE request.
- Middleware as a Gatekeeper
Middleware in Laravel works like a security checkpoint. It filters HTTP requests before they reach the application. By default, the ValidateCsrfToken middleware checks CSRF tokens for all incoming requests, ensuring no unauthorized access.
Middleware can also enforce other security measures, such as requiring HTTPS, managing sessions, or validating input data. This makes Laravel a powerful tool for building secure, user-friendly applications.
- Password Hashing and Encryption
A password is one of the most private pieces of user information, and Laravel takes security very seriously. Passwords are safely stored using the bcrypt hashing method, which renders them unchangeable and impervious to intrusions. Developers can connect passwords to Laravel’s authentication system and quickly hash them using the Hash::make method.
Laravel uses symmetric encryption with an application key to safeguard session and cookie data.
- Importance of the App Key
One of the main components of Laravel’s security architecture is the application key. It is essential for creating signed URLs, protecting sensitive data, and encrypting cookies. The php artisan key: create command should be used to produce this key during setup. Since encrypted data cannot be decrypted without it, it is essential to the security of your application.
Summing Up!
Laravel isn’t just a framework—it’s your go-to guide for building web apps that are fast, secure, and fun to create. With its clean structure, smart MVC design, and built-in security, Laravel takes the frustration out of coding so you can focus on what really matters: bringing your ideas to life.
Making your work easy is the main goal. Laravel is designed to help you stay focused and maintain the scalability of your apps, from its straightforward file structure to its versatile modular setup. Whether you are adding features, repairing problems, or maintaining security, see it as a well-maintained office where everything functions seamlessly.
Coding becomes an adventure rather than a work when using Laravel. Are you prepared to jump right in? Together, let’s make something truly remarkable!